In this post, I will explain to you how to leverage Google’s Mobile-Friendly Test tool in its API version with Python. As most of you must know, the Mobile-Friendly Test tool is a tool supplied by Google which indicates if a page is mobile-friendly or not. As well as indicating the mobile-friendliness from a page, the Mobile-Friendly Test tool also shows if there are resources that are blocked by Google and a screenshot from that page.
Taking into consideration that the Mobile-Friendly Test tool uses the Googlebot Smartphone user agent, it can be a very interesting tool to check how Google sees a page and if there is any problem with a resource that is blocked, apart from checking the mobile-friendliness of the page.
With the Mobile-Friendly Test tool API and Python, we can take this tool to the next level, being very useful to analyze in a bulk mode many pages and check how Googlebot sees them. Imagine that you make changes to your website layout, theme, or the way the pages of your website are served to the Googlebot. By using the Mobile-Friendly test tool API and Python you can check lots of pages very fast and easily to make sure that the Googlebot sees your website as expected by examining the blocked resources and the screenshots.
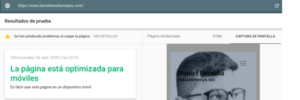
Table of Contents
Requirements and Assumptions
- Python 3 is installed and basic Python syntax is understood.
- Google API key has been set up.
- Google Search Console service is enabled.
Import Modules
We are going to use three Python modules to be able to make our requests to Mobile-Friendly Test tool API:
- requests – in order to make the request to the Mobile-Friendly Test tool API endpoint. We will send some parameters using a post method.
- json – in order to read and navigate through the JSON response.
- base64 – to download the screenshots as the API returns them encoded in base64.
The requests module must be installed. We can install it easily by using:
pip install requests
Having said this, let’s see how this API works!
Making the request to the API endpoint
The endpoint where we need to make the request is “https://searchconsole.googleapis.com/v1/urlTestingTools/mobileFriendlyTest:run” and we need to add as parameters:
- URL: the URL where we would like to make the request.
- requestScreenshot: if we would like to get the screenshot. The default value is false so if we want the screenshot to be returned we need to use “true”.
- key: the Google API key which has been set up previously.
url = 'https://searchconsole.googleapis.com/v1/urlTestingTools/mobileFriendlyTest:run' params = { 'url': 'https://www.danielherediamejias.com', 'requestScreenshot': 'true', 'key': "yourkey" } x = requests.post(url, data = params) data = json.loads(x.text)
In the code which is above we make our first request to the Mobile-Friendly Test tool API for the URL https://www.danielherediamejias.com. Once we get the response, we use the method json.loads() to read it as a JSON.
Which information can we get now?
- Response Code: important to make sure that the response code is 200. In case it is different, it could flag that the Googlebot smartphone has issues crawling that page.
- Status: if the audit has been completed or not.
- Mobile Friendliness “Verdict”: if the page is mobile friendly, it will just return: “MOBILE_FRIENDLY”. In the case of having mobile friendliness issues, then we will be able to get the specific issues too.
- Blocked resources: if there is any blocked resource, it is important to make sure that this blocked resource is not affecting Googlebot Smartphone in the way the page is displayed.
- Screenshot: in fact, we can get the screenshot and find out how Googlebot Smartphone is seeing our page.
The keys or variables that we would need to use to get the different resources explained above are:
- Response code: str(x)[len(str(x))-5:len(str(x))-2]
- Status: data[“testStatus”][“status”]
- Mobile Friendliness: data[“mobileFriendliness”]. If there are issues with some elements not being mobile friendly, we can get the list of issues with:
listmobileissues = [] for iteration in range (len(data["mobileFriendlyIssues"])): listmobileissues.append(data["mobileFriendlyIssues"][iteration]["rule"])
- Blocked Resources List:
listblockedresources = [] for iteration in range (len(data["resourceIssues"])): listblockedresources.append(data["resourceIssues"][iteration]["blockedResource"]["url"])
- Screenshot: data[“screenshot”][“data”]
Putting everything together
If we put everything together the code would look like this:
import requests import json import base64 try: url = 'https://searchconsole.googleapis.com/v1/urlTestingTools/mobileFriendlyTest:run' params = { 'url': 'yoururl', 'requestScreenshot': 'true', 'key': "yourkey" } x = requests.post(url, data = params) data = json.loads(x.text) with open("Finalscreenshot.png", "wb") as fh: fh.write(base64.b64decode(data["screenshot"]["data"])) print("Response code for Google Smartphone is " + str(x)[len(str(x))-5:len(str(x))-2]) print("Page is " + data["mobileFriendliness"]) if data["mobileFriendliness"] == "NOT_MOBILE_FRIENDLY": for iteration in range (len(data["mobileFriendlyIssues"])): print("The page has problems with " + str(data["mobileFriendlyIssues"][iteration]["rule"])) issues = data.get("resourceIssues", 0) if issues != 0: for iteration in range (len(data["resourceIssues"])): print("Problems with the blocked resources " + str(data["resourceIssues"][iteration]["blockedResource"]["url"])) print("Screenshot from " + str(params["url"]) + " is taken and downloaded.") except: print("Problem with " + str(params["url"]) + ". " + str(x)[len(str(x))-5:len(str(x))-2] + " Response Code.")
The output which this fragment of code will return is:
Plus in addition, it will download as a PNG image the screenshot which has been taken from the Mobile-Friendly Test tool. In my case the image I have gotten is:
In the case of having any issue with that page, then it will return a printed sentence that will indicate the response code which was gotten. If we also happen to run this piece of code with a not mobile-friendly page, we might get something like:
That is all, I hope that you liked this post and you found it useful!
Google Mobile Friendly Test API FAQ
- Using Google’s Mobile Friendly Test Tool API with Python - October 5, 2020